Summary
This blog contains a comprehensive description of various standard software architecture patterns, their importance, and the benefits they bring to your codebase.
What are Architecture Design Patterns and Why We Should Follow Them
Software Architecture Design Patterns are methodologies or sets of rules designed to isolate different components of your software, making it more readable, maintainable, and testable. These architectures primarily focus on separating your UI layer from your Business layer and Data layer.
UI Layer: This is typically the frontend of the software. It is the user interface layer with which the end user interacts. It encompasses everything the end user sees and interacts with, including the visual elements and user experience.
Business Layer: This layer manages and manipulates the core logic and data of the software. It encapsulates the business rules, processes, and operations that define how data can be created, displayed, stored, and changed.
Data Layer: Responsible for maintaining and storing data, this layer includes all the logic related to data management. It interacts with the database or any other data storage systems, handling data retrieval, storage, and update operations.
By adhering to these architecture design patterns, software development can achieve several benefits:
- Improved Readability: Clear separation of concerns makes it easier for developers to understand the structure and flow of the application.
- Enhanced Maintainability: Isolated components mean that changes in one part of the application have minimal impact on other parts, simplifying maintenance.
- Better Testability: With distinct layers, testing can be more focused and effective, allowing for individual layers to be tested in isolation.
- Scalability: Modular architecture patterns support the scalability of applications, enabling easier updates and expansions.
Let’s understand them one by one by considering a simple car clicker application which has the following features:
- Votes for the model of the car
- Name and image of the model
- When the image is clicked, the vote count increases
The different layer components are:
- UI: The list of the car models on the left, the car image, their name, and their count.
- Data: Vote count data of the car, names, and photos data of the car.
- Business Logic: How much vote increment should take place on click, what to do and how to do it when a certain element is clicked.
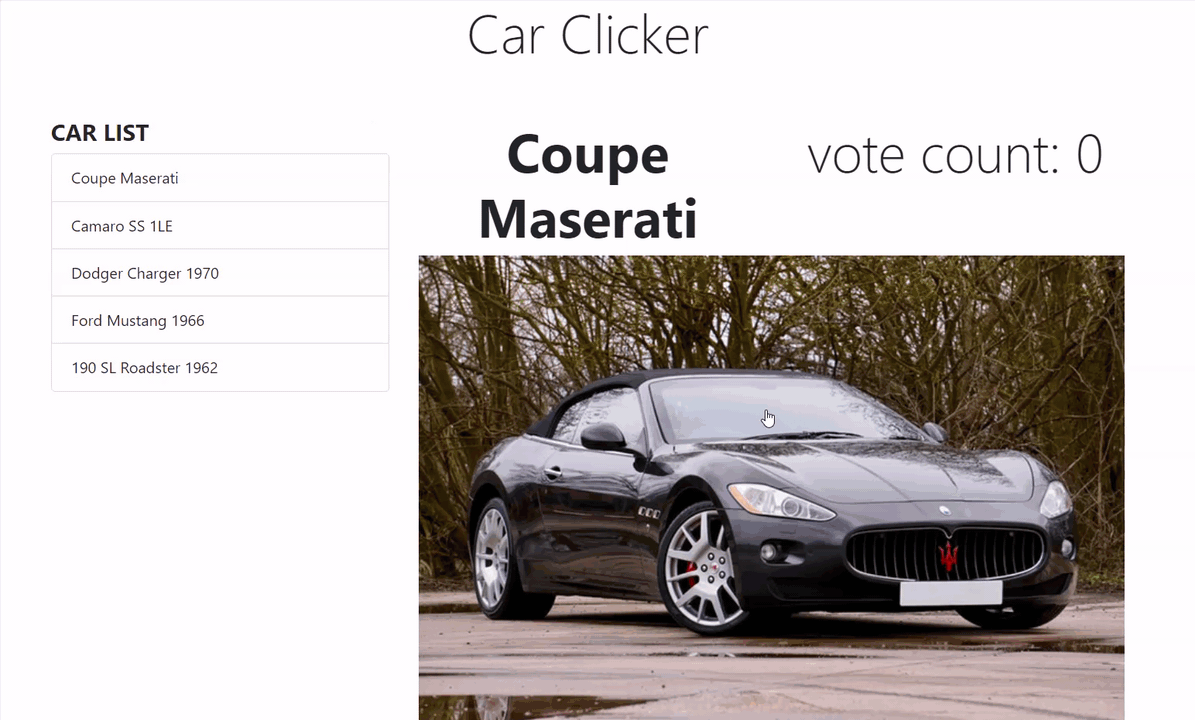
MVC (Model View Controller)
MVC stands for Model View Controller and divides the software into 3 parts named Model, View, and Controller as shown below:
- Model: This layer contains data logic and stores the data of the application and the logic related to it. So the model layer will be having the code to define schema, along with their getter and setter methods for different entities in the table.
- View: This layer contains the logic for the user interface. How to display the list of the car names, their vote count, and their image. View layer is also responsible to update itself and ask controller to update the model accordingly.
- Controller: The main brain of the software. This layer contains the core logic of what data needs to be sent to the View layer, how it needs to be updated/manipulated in the model layer.
Example: Car Clicker App (MVC)
- Model: Manages the car data (names, images, vote counts) and defines how to retrieve and update this data.
- View: Displays the car data (list of car models, images, vote counts) and updates the UI based on user interactions.
- Controller: Handles the logic of what happens when a car image is clicked (increments vote count), retrieves the updated data from the Model, and instructs the View to update the display.
MVP (Model View Presenter)
MVP stands for Model View Presenter and divides the software into 3 parts named Model, View, and Presenter as shown below:
- Model: Similar to MVC, this layer contains data logic and stores the data of the application and the logic related to it. It includes the schema definitions and getter/setter methods for the votes table and cars table.
- View: This layer contains the UI logic, defining how the car names, their vote counts, and images are displayed. However, in MVP, the View is more passive and delegates most of its responsibilities to the Presenter.
- Presenter: The Presenter acts as an intermediary between the View and the Model. It retrieves data from the Model, processes it, and updates the View. Unlike the Controller in MVC, the Presenter also handles the logic for updating the View in response to user interactions.
Example: Car Clicker App (MVP)
- Model: Manages the car data (names, images, vote counts) and defines how to retrieve and update this data.
- View: Displays the car data (list of car models, images, vote counts) but is mostly passive, waiting for the Presenter to update it.
- Presenter: Handles the logic of what happens when a car image is clicked (increments vote count), retrieves the updated data from the Model, and updates the View with the new data. The View notifies the Presenter of user actions, and the Presenter manages the response.
Difference Between MVC and MVP
Aspect | MVC | MVP |
---|---|---|
Controller/Presenter | Controller handles user input and updates Model and View | Presenter acts as an intermediary, handling logic and updates between Model and View |
View | View is more active, responsible for user interaction logic | View is more passive, primarily displaying data and delegating logic to Presenter |
Interaction | Direct interaction between View and Controller | View and Model interact only through the Presenter |
MVVM (Model View ViewModel)
MVVM stands for Model View ViewModel and divides the software into 3 parts named Model, View, and ViewModel as shown below:
- Model: This layer contains data logic and stores the data of the application and the logic related to it. It includes the schema definitions and getter/setter methods for the votes table and cars table.
- View: This layer contains the UI logic, defining how the car names, their vote counts, and images are displayed. In MVVM, the View is typically bound to properties and commands in the ViewModel.
- ViewModel: The ViewModel acts as a bridge between the View and the Model. It handles the presentation logic and state of the application, exposing data and commands to the View. It interacts with the Model to retrieve and update data, and provides data-binding mechanisms to the View.
Example: Car Clicker App (MVVM)
- Model: Manages the car data (names, images, vote counts) and defines how to retrieve and update this data.
- View: Displays the car data (list of car models, images, vote counts) and updates the UI based on the data and commands from the ViewModel.
- ViewModel: Handles the logic of what happens when a car image is clicked (increments vote count), retrieves the updated data from the Model, and updates the View with the new data using data-binding. The ViewModel exposes properties and commands that the View binds to, facilitating interaction without direct communication.
VIPER (View Interactor Presenter Entity Router)
VIPER stands for View Interactor Presenter Entity Router and divides the software into 5 parts named View, Interactor, Presenter, Entity, and Router as shown below:
- View: This layer contains the UI logic and is responsible for displaying data and interacting with the user. It receives updates from the Presenter and sends user actions to the Presenter.
- Interactor: This layer contains the business logic and interacts with the Entity layer to fetch or persist data. It communicates with the Presenter to send back data. It generally contains the chore business logic and developers prefer it as it neither makes your make your model fat, nor your model
- Presenter: The Presenter acts as an intermediary between the View and the Interactor. It handles the presentation logic and prepares data for display in the View. It receives user actions from the View and triggers the Interactor to perform business logic.
- Entity: This layer contains the data models and manages data structures and schema definitions. It represents the business data that the application works with.
- Router: This layer handles navigation and routing logic. It manages the flow of screens and passes data between different parts of the application.
Example: Car Clicker App (VIPER)
- View: Displays the car data (list of car models, images, vote counts) and updates the UI based on user interactions.
- Interactor: Handles the logic of what happens when a car image is clicked (increments vote count), retrieves the updated data from the Entity, and sends it to the Presenter.
- Presenter: Retrieves data from the Interactor, processes it, and instructs the View to update the display. It handles the logic for updating the View in response to user interactions.
- Entity: Manages the car data (names, images, vote counts) and defines how to retrieve and update this data.
- Router: Manages navigation between different parts of the application, ensuring that the correct data is passed along.
Difference Between MVC, MVP, MVVM, and VIPER
Aspect | MVC | MVP | MVVM | VIPER |
---|---|---|---|---|
Controller/Presenter/ ViewModel/Interactor | Controller handles user input and updates Model and View | Presenter acts as an intermediary, handling logic and updates between Model and View | ViewModel acts as a bridge, exposing data and commands to the View | Interactor handles business logic and interacts with Entity |
View | View is more active, responsible for user interaction logic | View is more passive, primarily displaying data and delegating logic to Presenter | View binds to ViewModel properties and commands, facilitating interaction | View is passive, only displaying data and sending user actions to Presenter |
Interaction | Direct interaction between View and Controller | View and Model interact only through the Presenter | View and Model interact through data-binding with the ViewModel | View interacts with Presenter, which communicates with Interactor and Entity |
Navigation | Handled by Controller | Handled by Presenter | Handled by ViewModel or external mechanisms | Handled by Router, separating navigation logic |
My Opinion
Personally, I feel VIPER isolates different section of your logic in the most accurate way such that all the layers work independently and can be scaled and maintained easily. It also solves the Fat Controller Thin Model (Like in MVC and MVP) problem along with Thin Controller Fat Model (Like in MVVM) problem by separating out the business logic into completely different layer.
Remember to check back next week for an amazing blog post!