Summary
Everyone talks about server security, but very few are actually knowledgeable about it. There could be multiple vulnerabilities with your backend application, and there are very high chances that you might not know about them. This article discusses multiple basic methods of securing your backend application along with the most common vulnerabilities your server could be exposed to.
Authentication and Authorization Mechanisms
Authentication and authorization are crucial for ensuring that users are who they say they are and that they have permission to access the requested resources.
- Authentication is the process of verifying the identity of a user.
- Use strong password policies.
- Implement multi-factor authentication (MFA).
- Use OAuth, JWT, or other secure token-based systems.
- Authorization determines what an authenticated user is allowed to do.
- Implement role-based access control (RBAC).
- Ensure proper permission checks on every request.
By ensuring robust authentication and authorization mechanisms, you can significantly reduce the risk of unauthorized access to your backend systems.
Securing Data in Transit and at Rest
Data security involves protecting data from unauthorized access and corruption throughout its lifecycle.
Data in Transit:
- Use HTTPS to encrypt data transmitted over the network.
- Employ secure communication protocols like TLS.
- Validate SSL certificates.
Data at Rest:
- Encrypt sensitive data stored in databases.
- Use encryption keys and manage them securely.
- Regularly back up data and ensure backups are also encrypted.
Securing data both in transit and at rest ensures that sensitive information remains confidential and integral, even if intercepted or accessed by unauthorized parties.
Protecting Against Common Vulnerabilities as Identified by the OWASP (Top 10)
The OWASP Top 10 is a standard awareness document for developers and web application security. It represents a broad consensus about the most critical security risks to web applications.
- Injection: Ensure to use prepared statements and parameterized queries.
- Broken Authentication: Implement strong authentication mechanisms.
- Sensitive Data Exposure: Encrypt sensitive data and ensure secure data transmission.
- XML External Entities (XXE): Disable DTDs and external entities in XML parsers.
- Broken Access Control: Enforce strict access control policies.
- Security Misconfiguration: Regularly update and patch systems.
- Cross-Site Scripting (XSS): Sanitize and validate all user inputs.
- Insecure Deserialization: Use secure deserialization methods and validation.
- Using Components with Known Vulnerabilities: Regularly update and patch third-party libraries.
- Insufficient Logging and Monitoring: Implement robust logging and monitoring mechanisms.
Understanding and mitigating the OWASP Top 10 vulnerabilities is fundamental to building a secure backend application.
Denial of Service (DoS)
A Denial of Service attack aims to make a server or network resource unavailable to its intended users by overwhelming it with a flood of illegitimate requests.
- Rate Limiting: Implement rate limiting to restrict the number of requests a user can make in a given time period.
- Traffic Filtering: Use firewalls and traffic filtering mechanisms to block malicious traffic.
- Load Balancing: Distribute traffic across multiple servers to handle high loads.
- DDoS Protection Services: Utilize services like Cloudflare or AWS Shield to mitigate large-scale attacks.
Protecting against DoS attacks involves a combination of traffic management, resource allocation, and specialized mitigation services.
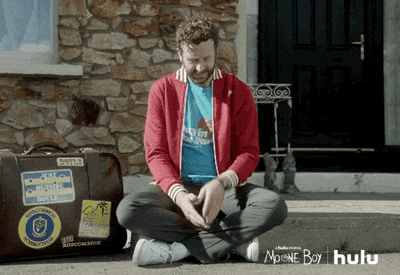
Code Injection
Code injection occurs when an attacker is able to introduce malicious code into a vulnerable program, potentially leading to a wide range of security issues.
- Input Validation: Always validate and sanitize user inputs.
- Use Prepared Statements: Employ prepared statements for database queries.
- Avoid Dynamic Queries: Refrain from using dynamic queries that concatenate strings.
- Code Review and Testing: Regularly conduct code reviews and automated testing to identify and fix vulnerabilities.
By adhering to best practices for input validation and query handling, you can significantly reduce the risk of code injection attacks.
References
Ensuring the security of your backend application is a continuous process that requires vigilance and adherence to best practices. By implementing robust security measures, you can protect your systems from a wide range of threats and vulnerabilities.